I recently had a need in an application to have an “In Memory Queue”. Simply put, a list of items that I add to, and I can request the oldest items out of the queue. It’s a very common construct in other programming languages/platforms such as Java or C#.
Unfortunately, Javascript does not have native queueing functionality, and so I had to build my own. What I found however, is that Javascript itself actually has all the pieces of the puzzle to make this a real breeze.
First I created a simple Queue class. Because I am using Typescript in Angular, I could use generics to say which type of items my queue would hold. The first thing I add to my class is an array of items of T (My type), that I then initialize to being empty.
export class Queue<T> {
items : T[] = [];
}
My enqueue method is easy. I use the native javascript function of “push” on an array to add items to the end. Notice also that I specify the item being added is of type T.
enqueue(item : T) : void {
this.items.push(item);
}
Then for my dequeue, I can use the javascript function of “shift” which essentially pulls items from the *front* of the array. This is important because it means we are adding to the back, but pulling from the front, much like how a queue works. Also important to note is that when we call shift, if the array is empty, it will return undefined.
dequeue() : T {
return this.items.shift();
}
Next I wanted to add a couple more helpers such as peeking at messages (Reading the next message off the queue without actually removing it), and knowing if the queue is empty. So the class in it’s entirety looked like so :
export class Queue<T> {
items : T[] = [];
enqueue(item : T) : void {
this.items.push(item);
}
dequeue() : T {
return this.items.shift();
}
peek() : T {
if(this.items.length) {
return this.items[0];
} else {
return undefined;
}
}
isEmpty() {
return !this.items.length;
}
}
Then we just use our class :
let myQueue = new Queue<number>();
myQueue.enqueue(1);
myQueue.enqueue(2);
myQueue.enqueue(3);
let nextItem = myQueue.dequeue(); //Dequeues the number 1
Is this the most performant code in the world? Absolutely not. But using existing javascript language features, we created a queue in no time at all!
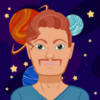
💬 Leave a comment