When strict mode is enabled in our React application it runs extra checks in the background looking for warnings. It’s a great tool for highlighting issues in applications. It’s quite similar to React Fragment in that it doesn’t render any extra DOM elements or UI.
StrictMode usage
If you have used the
$ npx create-react-app myapp
command to set up your project, then the strict mode is already enabled by default in your application.
It’s extremely simple to enable React strict mode in your application. All you have to do is to import the component and use it within your application.
import React, { StrictMode } from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
ReactDOM.render(
<StrictMode>
<App />
</StrictMode>,
document.getElementById('root')
);
It’s simple as that! Just make sure that you use the StrictMode component at the top of your DOM structure to make sure that it encompasses all of the components.
Now that you have enabled the strict mode, you may want to refresh your application and check out the console section of the developer tools to check if it detected something.
As of the current version, it helps with:
- Spotting unsafe component lifecycles
- Identifying the deprecated methods
- Detecting unexpected side effects
Official docs for React describe strict mode as a tool that’s meant to be used only in the development mode, so if you’re working on a live application you shouldn’t be worried about introducing some unwanted behaviour to your application.
Take a look at some example warnings:


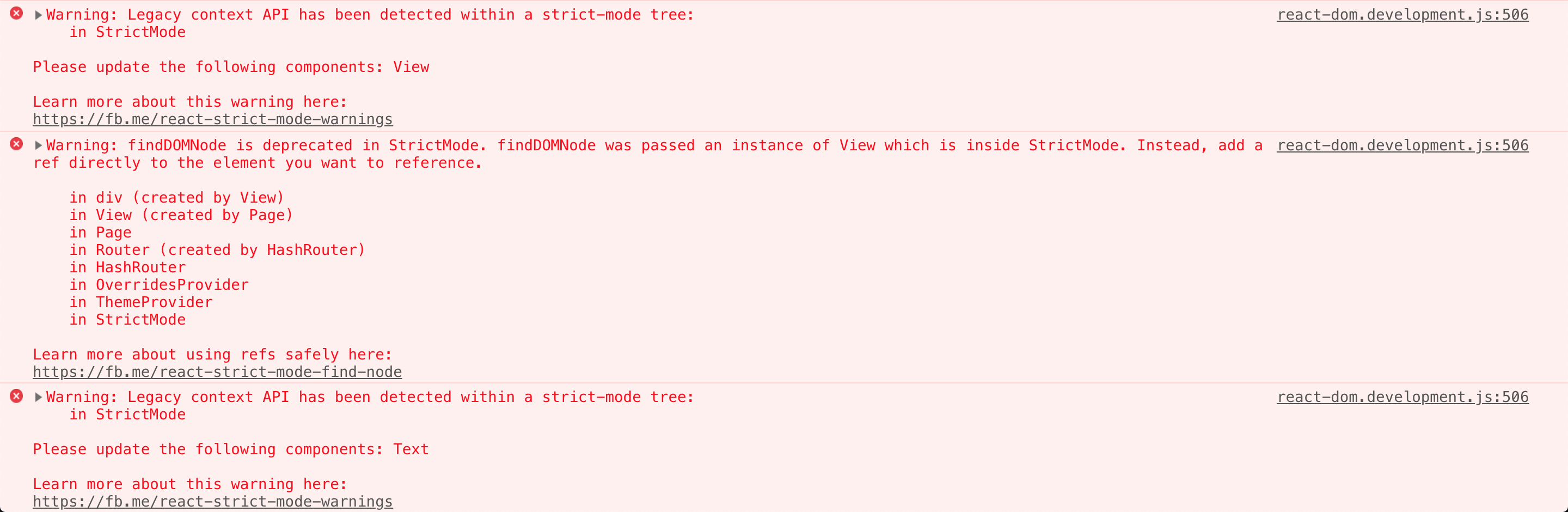
It’s worth keeping in mind that strict mode is still being developed by the React team so it will only keep on getting better and better.
๐ฌ Leave a comment