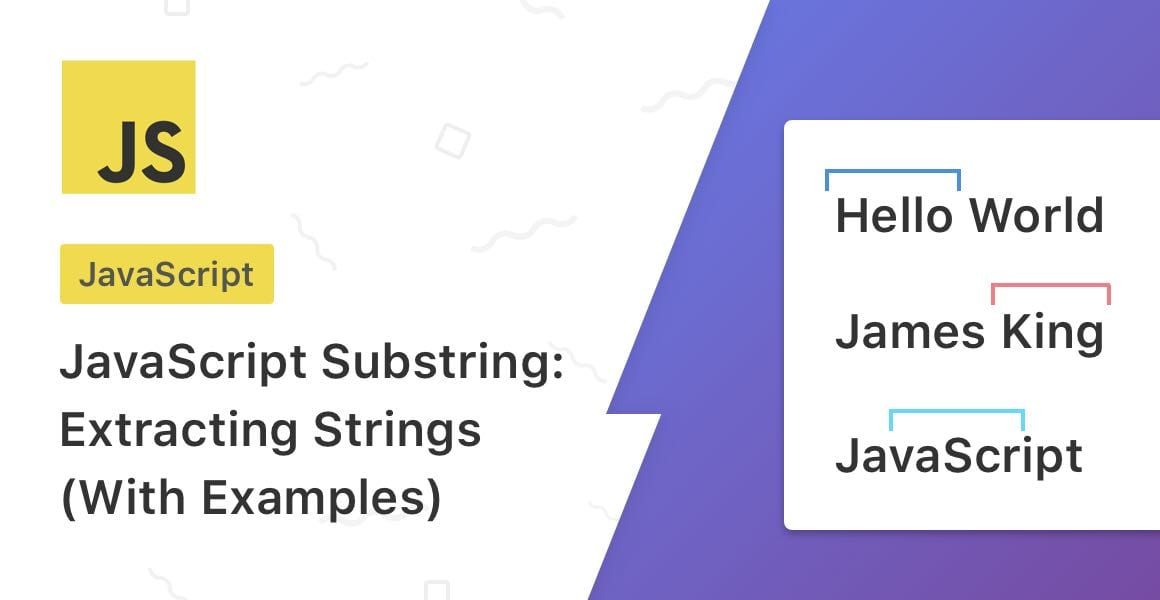
The JavaScript substring method returns part of a string that is specified through two parameters: the start character and end character of that string.
What is the Substring Method?
string.substring(startIndex, endIndex);
Substring in JavaScript is a method that allows us to select a specific part of a string.
The substring method takes two arguments:
- The start index of the character of the string to start extracting (Tip: this includes spaces!)
- The end index of the character of the string to stop extracting (again, includes spaces) This is optional
Substring does not modify the original string.
How to use Substring in JavaScript
const name = "James King";
const firstName = name.substring(0, 5);
The result of firstName is James. Let’s find out why.
The example above has us declaring a string constant, name, and its value is a string of my full name.
We then declare another constant named firstName and assign it the value of characters 0 – 5 of name. Beginning from 0 (as all indexes in JavaScript do), we count 5 characters from left to right of name.
Substring selects the characters between the specified index arguments. The end index value is not included in the returned string from substring. Therefore, “dog”.substring(0,1) will return only ‘d’.
As a result, that’s what will be returned from the substring method and assigned to the firstName constant.
Example: Return All Characters After a Character in a String
const todo1 = "01 - Pick up dry-cleaning";
const todo2 = "02 - Go food shopping";
const todo1Task = todo1.substring(5);
const todo2Task = todo2.substring(5);
The example above shows how you can return the rest of the string after a specified starting index.
This is good if your strings are all formatted in a certain way, or you don’t know how long your strings are, or you have multiple strings that are all different lengths.
Remember, the second argument in the JavaScript substring method is optional.
JavaScript Substring from the End of a String
Let’s explore how to remove characters from the end of a string using a reverse index.
const desc = "Upmostly teaches you React and JavaScript";
const justJavaScript = desc.substring(desc.length - 10, desc.length);
The example above demonstrates how we can select the last word, “JavaScript”, in the full desc string.
We pass in the length of the whole string minus 10 characters, which is the length of the string that we want to extract. The second argument takes the whole length of the string as we want to end at the very last character of it.
When to Use Substring in JavaScript
Strings are probably the most common type to work with in JavaScript. (unless you’re building a calculator or something that is heavily numbers based), and therefore you’ll be manipulating strings often.
Once you begin pulling data from outside sources, such as databases and APIs) into your JavaScript application, you’ll find yourself doing more and more string manipulation.
You never know how databases or API responses are structured, and therefore the data response may not be exactly how you need it to be in your JavaScript app. The database may not separate a person’s name into firstName and lastName, therefore you’ll need to separate them yourself using substring.
To continue learning more about String methods in JavaScript, read how to remove a character from a string in JavaScript.
💬 Leave a comment