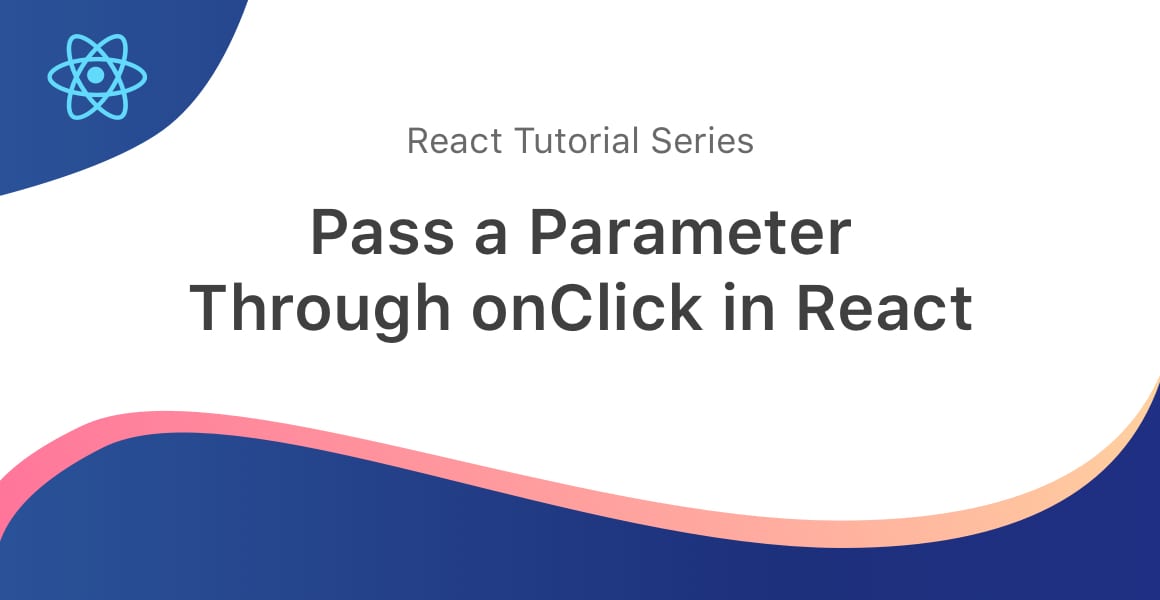
A common thing you will want to learn in React is how to pass a value as a parameter through the onClick event handler. Read on to learn how!
import React from 'react';
const ExampleComponent = () => {
function sayHello(name) {
alert(`hello, ${name}`);
}
return (
<button onClick={() => sayHello('James')}>Greet</button>
);
}
export default ExampleComponent;
For those who want the TL;DR and go straight to the code, take a look at the example above ☝️.
ExampleComponent is a very simple React component that contains one button and one function.
To understand how to pass a value as a parameter through an onClick event handler, take a look at the line of code inside of the return statement. It’s a single button with one event handler: onClick.
Typically, to call a function when we click a button in React, we would simply pass in the name of the function to the onClick handler, like so:
...
return (
<button onClick={sayHello}>Greet</button>
);
...
Notice how in the ExampleComponent code above, we pass in more than just the function name.
In order to pass a value as a parameter through the onClick handler we pass in an arrow function which returns a call to the sayHello function.
In our example, that argument is a string: ‘James‘:
...
return (
<button onClick={() => sayHello('James')}>Greet</button>
);
...
It’s this trick of writing an inline arrow function inside of the onClick handler which allows us to pass in values as a parameter in React.
Let’s explore some more common examples!
Pass a Button’s Value as a Parameter Through the onClick Event Handler
You might want to pass in the value or name of the button or input element through the event handler.
...
return (
<button value="blue" onClick={e => changeColor(e.target.value)}>Color Change</button>
);
...
The button now has an additional attribute, named value. We can get access to the button’s attributes in its event handlers by accessing the event through the event handler.
The example above shows the variable e that is provided from the onClick event handler. This stands for event. Once we have the event, we can access values such as the value or name attribute.
To learn more about the onClick event handler read my tutorial on the onClick Event Handler (With Examples).
💬 Leave a comment