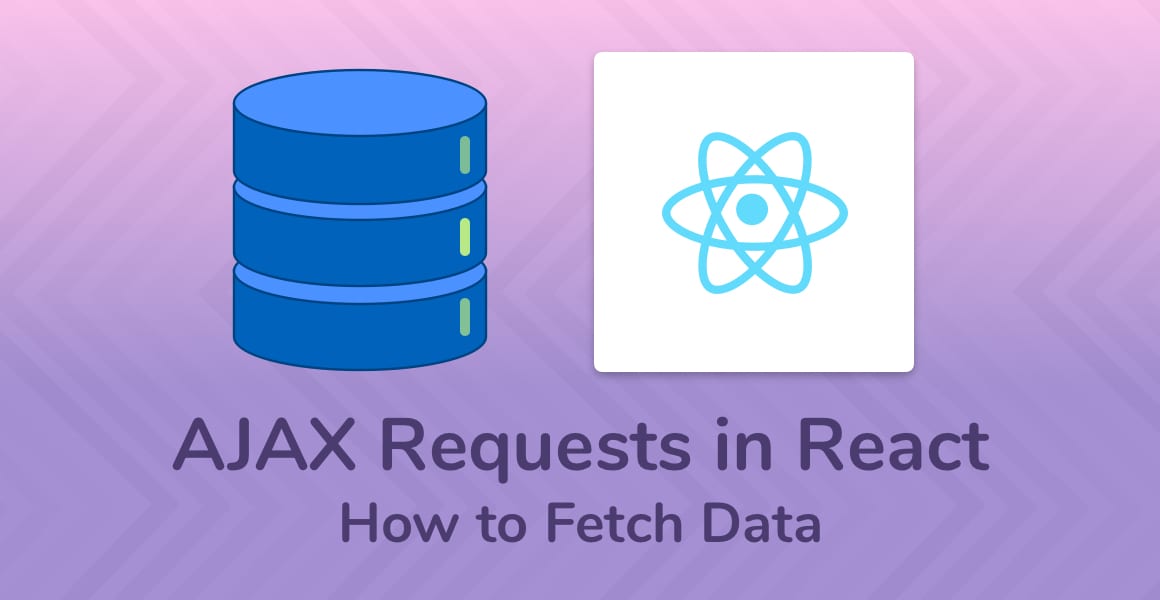
Sooner or later, every React developer needs to know how to make AJAX requests to fetch data from a server (make API calls). Let’s learn how!
TL;DR
async function getUsers() {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
const users = await response.json();
}
Today, I’ll show you how to:
- Choose and set up an HTTP library to make AJAX requests in your React components (Using React Hooks!). You can learn more about React Hooks from this guide
- Make a GET and POST HTTP request to an API.
- Display the data returned in the API response in a React component.
Choosing an HTTP Library to Make AJAX Requests
React is a front-end library, therefore it has no opinion on how you fetch data. React is only interested in how to display data.
To make AJAX requests and fetch data from a server, you need an HTTP library.
As always with web development, there are a million choices when it comes to picking a tool for the job.
As a result, making AJAX requests are no exception. There are two popular libraries for making HTTP requests in JavaScript: Axios and SuperAgent.
There’s also the Fetch API, which is a standard API supported in all modern web browsers. Therefore, it doesn’t require you to install a third party library.
Decisions, decisions! I’m going to make your lives easier and choose for you so your heads don’t explode — we’re going with Fetch for now, but feel free to explore the other two later.
If you’re interested in Axios, check out my tutorial on Using Axios to Make API Requests in React.
A Simple AJAX in React Example
We’ll start with an example of a very simple React component that, on render, makes an AJAX request to get a list of users.
import React, { useEffect, useState } from 'react';
const User = () => {
const [users, setUsers] = useState([]);
useEffect(() => {
getUsers();
}, []);
async function getUsers() {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
const users = await response.json();
setUsers(users);
}
return (
<ul>
{users.map(user => (
<li>{user.name}</li>
))}
</ul>
);
};
export default User;
I’m using the excellent JSONPlaceHolder API, which is a fake API for testing and prototyping. Perfect for when we’re making AJAX calls to bring the data into React!
I’m using the latest version of React, which introduced React Hooks. The component in the example is a stateful functional component which uses the useState Hook (No need to write Class components anymore). If you want to learn more about how the useState hook works check out this guide
If you haven’t yet explored Hooks, I urge you to read A Simple Introduction to React Hooks.
async function getUsers() {
const response = await fetch('https://jsonplaceholder.typicode.com/users');
const users = await response.json();
setUsers(users);
}
This function is where the magic happens. It makes an AJAX request within our React component to the API to get a list of users, sets the response to a users variable, and then stores the users in the component’s state.
A few more interesting points about the function above:
- It uses the async/await syntax. This is much more readable than using promises.
- The code will stop at the await keyword and wait for the AJAX request to come back with a response.
- It’s an asynchronous function, meaning it runs the code and doesn’t stop the rest of the app from working.
Making a POST AJAX Request
Making a POST request is a little different to a GET request. A POST contains a body of data that is sent from our end, the React component, to the server.
async function createUser(body) {
const response = await fetch('https://jsonplaceholder.typicode.com/users', {
method: 'POST',
body: JSON.stringify(body),
headers: {
"Content-type": "application/json; charset=UTF-8"
}
});
}
The createUser function above takes one parameter, the data to be sent in the AJAX requests body. The URL remains the same, we’re not changing that. We’re only changing the method from a GET request to a POST request.
Finally, we Stringify (basically, convert it to a string) the body object so that we can send it as a JSON string to the web server for the API to understand and parse it correctly.
Wrapping Up
Now you know how to make AJAX requests in React components, you’re on your way to building some truly amazing web apps!
If you enjoyed this tutorial, why not sign up to the Upmostly Newsletter? I write one tutorial per week and at the end of the month, bundle them all into one awesome email for my subscribers to read.
The Newsletter sign up is just a short scroll away.
As always, leave a comment if you enjoyed the tutorial or if you have a question. I’m always around to answer!
💬 Leave a comment