One of the key parts of any application is the build process – using your code to build together something that can be ran. For Next.js, this means things like optimising your site’s assets, transforming TypeScript code into JavaScript, and minifying your code.
This leaves us with an output build folder, ready to be deployed wherever you need. In short, in Next.js by default your build folder is in “.next” in your project folder, but if you’re interested, in this article we’ll talk you through building your app, and configuring your build folder.
Build
To build your Next.js project you need to run the build command:
npm run build
in the root directory of your project. By default, this will generate the .next folder.
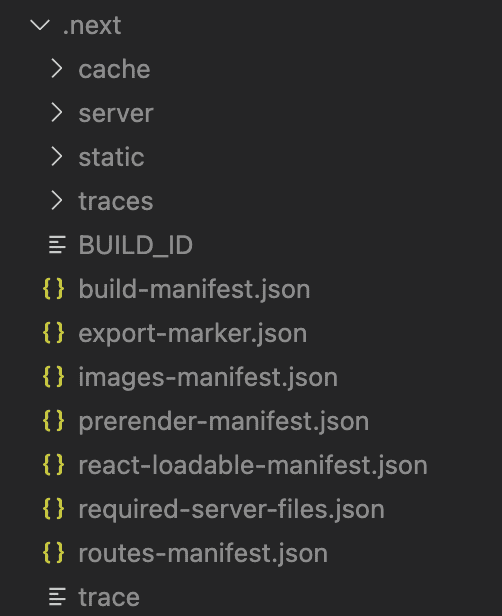
All of the code and files located within that folder has been processed specifically to allow browsers use it more efficiently.
Configuration
Changing the location of your build folder is simple, and can be done through your Next.js config file, inside “next.config.js”. You can change the build location through the “distDir” option. Here’s an example Next.js config, but if you’re working with an existing one, all you need to do is add in the distDir option with your folder of choice.
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
distDir: 'dist'
};
module.exports = nextConfig;
One key thing to note is that this folder should be within your project, i.e. distDir could not be something like “../../dist”
Export
Next.js also supports exporting your app as a static site through the config file, through the output property:
/** @type {import('next').NextConfig} */
const nextConfig = {
reactStrictMode: true,
distDir: 'dist'
output: 'export
};
module.exports = nextConfig;
If you are using this feature, for example to deploy your app through GitHub Pages then the default output directory for your app will be “out”. You can still change this, however. If you have a build folder option set in your Next.js config, then this option will take precedence.
Conclusion
And that’s all, thanks for reading! Hopefully with this article, I was able to answer any questions you have on where your build folder is located in your Next.js app. If you liked this article, or if you have any further questions, feel free to leave a comment below.
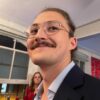
💬 Leave a comment