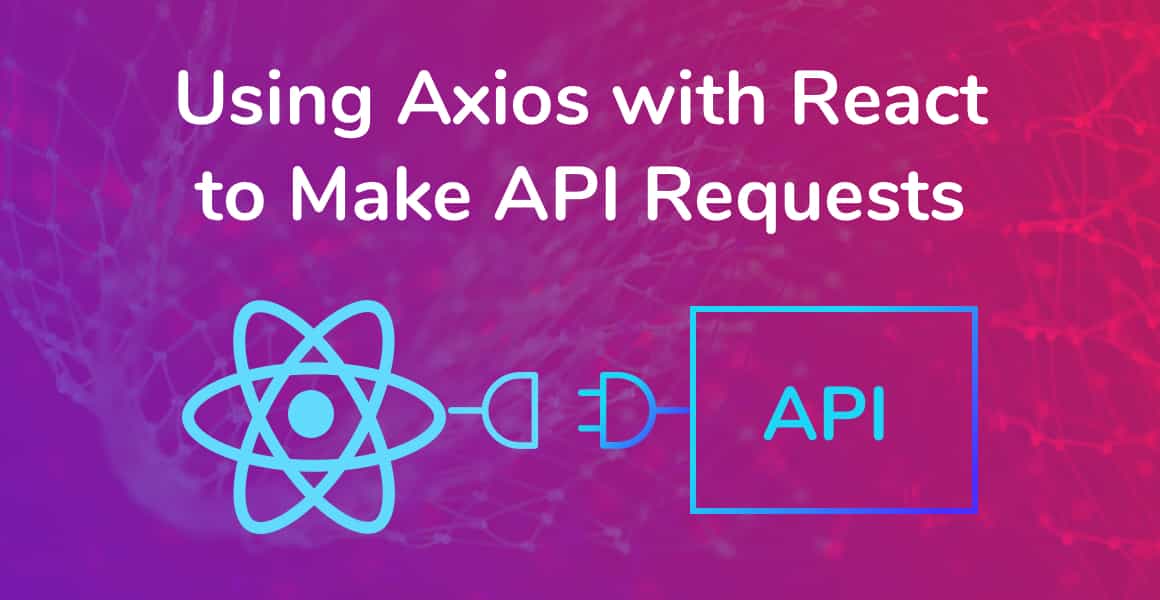
Axios is a library used to make HTTP requests from the browser. Read on to learn how to use Axios with React to make API requests and display the response.
What is Axios?
Axios is an extremely popular (over 52k stars on Github) HTTP client that allows us to make GET and POST requests from the browser.
Therefore, we can use Axios with React to make requests to an API, return data from the API, and then do things with that data in our React app.
Why Do We Need Axios?
TL;DR: Axios allows us to communicate with APIs easily in our React apps.
Most web and mobile apps store data in the cloud or communicate with a service. For example, a service that gets the current weather in your local area, or returns a list of GIFs based on a search term.
Databases and web services have something called an API (Application Programming Interface) which apps can use to communicate with through a URL. In other words, an API allows apps to retrieve or send data to and from databases or web services.
Apps use HTTP requests, for example, GET, POST and PUT, to communicate with APIs. In short, Axios make it easy for our apps to perform these commands.
To learn more about fetching data from an API in a real application, check out our tutorial on building a complete React app with Airtable.
Installing Axios
To use Axios with React we need to install Axios. If you’re using NPM, open up a new terminal window, get to your project’s root directory, and run the following to add Axios to your package.json.
npm i axios --save
Open up App.js and import the Axios library at the top of the file.
...
import axios from 'axios';
...
Retrieving Data with a GET Request
The most common type of HTTP request is the GET request. It allows us to retrieve data from an API and use that data in a React app.
There is plenty of free and open APIs to use. For this tutorial, we’ll use the Dog API, the internet’s largest collection of dog photos!
Although this API sounds trivial, the data response is very simple, therefore it’ll be nice and easy to understand how to use the data response in our React app.
Let’s make our first API call to the Dog API using Axios.
To App.js, add a componentDidMount method, and then add the following code:
...
componentDidMount() {
axios.get('https://dog.ceo/api/breeds/image/random')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
});
}
...
What’s happening above:
- We make a GET request to the Dog API using axios.get
- The Dog API documentation tells us that making a GET request to https://dog.ceo/api/breeds/image/random will give us a random dog photo.
- We then output the API data response to the browser’s console, using console.log.
Save your file, hop on over to your React app running in your browser and open up the developer console.
You should see the following response, although your message will have a different value as we’re asking for random photos each time.
{
status: "success",
message: "https://images.dog.ceo/breeds/pembroke/n02113023_3167.jpg"
}
Great! You’ve made your first API call using Axios with React. Now let’s do something useful with that response.
Using the API Data Response in React
API calls are made asynchronously because we have to wait for the server to return the data to the app. In other words, once an API call has been made, there may be a few seconds of wait time before the API returns data.
To show the photo of the dog in our React app, we need to store the API response in state.
Add a constructor, with an initial state property called imageURL:
...
constructor(props) {
super(props);
this.state = {
imageURL: '',
}
}
...
This is how we initialize state in class-based component in React. If you want to learn about using state in functional components check out this guide and this guide to learn about the other differences between Functional and class-based components. Now that we have initialized the state, let’s update the state when the API request returns data successfully.
...
componentDidMount() {
axios.get('https://dog.ceo/api/breeds/image/random')
.then(response => {
this.setState({ imageURL: response.data.message });
})
.catch(error => {
console.log(error);
});
}
...
We set the imageURL to be response.data.message because the image URL of the dog is found in the message key in the API response.
Always read API documentation. Although good APIs should stick to good RESTful design standards, they will always be different. The best way to know how the data response is structured is to read the documentation.
Finally, bring the imageURL state property into the render method, add an image element and set the image’s src to be the imageURL.
...
render() {
const { imageURL } = this.state;
return (
<img src={imageURL} />
);
}
...
Save your file, jump back over to the app in your browser and watch a random photo of a dog show up each time you refresh!
If requesting photos of dogs from an API isn’t what the internet was created for, I don’t know what is.
Wrapping Up
I hope you had fun learning about using Axios with React, making API requests and building a data-driven app! If you have any questions, leave a comment below and I’ll help you out.
See you next time!
💬 Leave a comment