Ref means just reference, so it can be a reference to anything (DOM node, Javascript value, etc). The useRef hook returns a mutable object which doesn’t re-render the component when it’s changed. Think it like useState, but unlike useState, doesn’t trigger re-render of the component. The object that useRef returns have a current property that can hold any modifiable value.
Syntax:

The initialValue
is the initial .current
property.
For example:
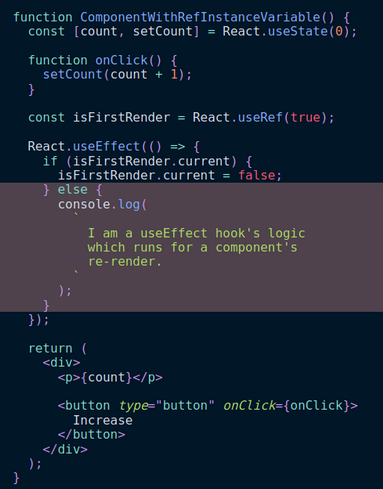
In the above code example, in the first render, the ref’s current property was true, so after first render when useEffect starts, it makes current property false. But if we click one more time, now useEffect can’t change current since it is false, but in the else block, we can run whatever we want. In this way, we can accomplish to use useEffect in every update but not the first time.
Rule of thumb: Whenever you need to track state in your React component which shouldn’t trigger a re-render of your component, you can use React’s useRef Hook to create an instance variable for it.
Now we came to React’s ref specialty, the DOM:
refs are primarily considered as a way to access the DOM nodes or react elements. If we pass a ref object to React with <div ref={myRef} />
, React will set its .current
property to the corresponding DOM node whenever that node changes.
Example:
function Form () {
const nameRef = React.useRef()
const emailRef = React.useRef()
const passwordRef = React.useRef()
const handleSubmit = e => {
e.preventDefault()
const name = nameRef.current.value
const email = emailRef.current.value
const password = passwordRef.current.value
console.log(name, email, password)
}
return (
<React.Fragment>
<label>
Name:
<input
placeholder="name"
type="text"
ref={nameRef}
/>
</label>
<label>
Email:
<input
placeholder="email"
type="text"
ref={emailRef}
/>
</label>
<label>
Password:
<input
placeholder="password"
type="text"
ref={passwordRef}
/>
</label>
<hr />
<button onClick={() => nameRef.current.focus()}>
Focus Name Input
</button>
<button onClick={() => emailRef.current.focus()}>
Focus Email Input
</button>
<button onClick={() => passwordRef.current.focus()}>
Focus Password Input
</button>
<hr />
<button onClick={handleSubmit}>Submit</button>
</React.Fragment>
)
}
Output:
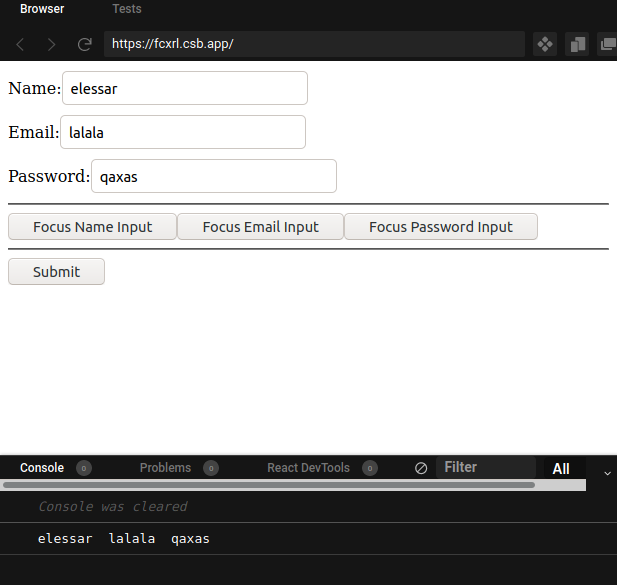
When clicked email , it’ll focus on email input:
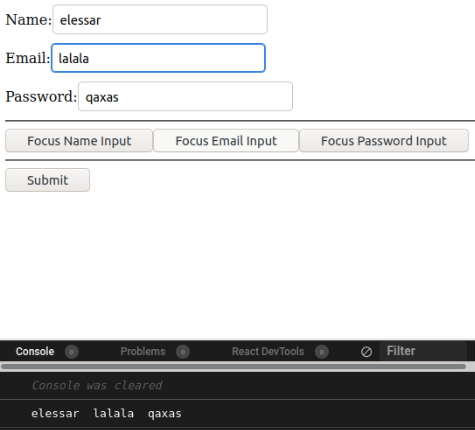
And that’s it for this article. I hope you enjoyed and learned a lot.
💬 Leave a comment