Introduction
If you’ve just started working with React or you’ve just started planning on how you may tackle learning it, you’ve surely bumped into plenty of new concepts such as State, Lifecycle Methods, Props, Hooks, Functional and Class Components, as well as many others.
If that might be the case, I would strongly recommend checking out this article, which talks about React State & the useState Hook, as well as this article, which provides an insightful overview of what React Hooks are.
And with that said, today we’ll be diving into a couple of those concepts by going more in-depth in order to learn more about one of the most used hooks that React has to offer: The useEffect hook.
Overview of the useEffect Hook
The useEffect Hook is React’s main approach to handling Side Effects.
What are Side Effects?
Side Effects refer to anything that affects something outside the scope of the function being executed.
Data fetching, setting up a subscription, and manually changing the DOM in React components are all examples of side effects. Whether or not you’re used to calling these operations “side effects” (or just “effects”), you’ve likely performed them in your components before.
React’s Official Docs
Side Effects are not specific to React per se, it is a general concept about behaviors of functions. A function is said to have side effects if it tries to modify anything outside its body.
That’s the reason for which the useEffect hook accepts a callback function as its first argument that will run every time there’s a re-render in the component’s state by default (Which means that whenever any of the state or props of the component change, the useEffect‘s callback will be executed). This behavior can, however, be overridden by providing a Dependency Array as a second argument.
The Dependency Array can either be empty, which will cause the callback function to be invoked only once when the component has first mounted, or it may contain state variables, which will cause the callback passed to the useEffect hook to be executed each time any of the state variables‘ value has changed.
Furthermore, the useEffect hook’s callback might also return a function that will be executed once the component is to unmount. That can either happen due to any conditional logic on which the component is being rendered, navigating away from the page where the component is, or closing the Browser Tab.
Usage of the useEffect Hook
Example Overview
Let’s say we want to have a simple counter component where a user will be presented with a button that he can click in order to increment a counter initially set to 0. We’ll display the counter value above the button, as well as in the title of the Browser Tab.
This example requires us to use an useEffect hook in order to track the value of the counter and update the title of the Browser Tab accordingly.
When the counter reaches 10 we’ll unmount the component and show a “Bye-bye” message to the user, to indicate that he has pressed the button enough times.
That will be achieved by a state variable that holds a boolean value and will be used for the conditional logic, as well as by using the return statement of the useEffect hook’s callback to handle the message when the component unmounts.
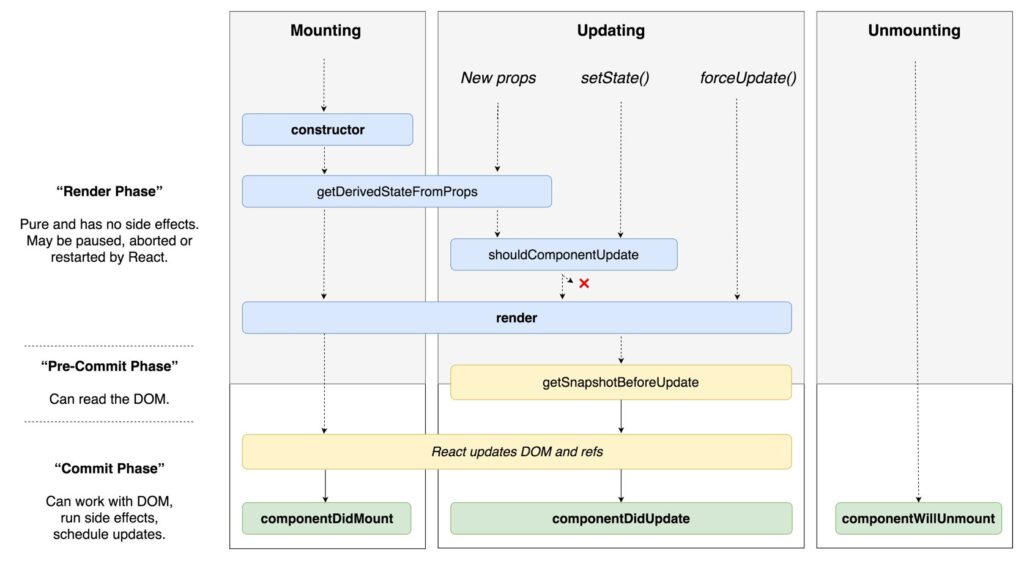
Code Overview & Explanation
We’ll start by creating a new Counter component under src/components/Counter/index.js
where we’ll put the logic of the counter component:
import { useEffect } from "react";
const Counter = ({ count, increment }) => {
useEffect(() => () => {
document.title = 'Counter';
alert('Hopefully you\'ve enjoyed our application!');
}, []);
return (
<div className="counter">
<p>Current Count: {count}</p>
<button onClick={increment}>
Increment Count
</button>
</div>
);
};
export default Counter;
It’s all rather straightforward; we’re rendering a button and a count within an HTML paragraph element and both interact with the counter state (count) and a wrapper around the counter setter method (the increment method).
Apart from the state interaction, we’re also using an useEffect hook to react to the unmount event; an event that’s going to be handled by updating the title of the Browser Tab and sending an alert to our users.
Now let’s see how our src/App.js
file looks like:
import { useState, useEffect } from 'react';
import './App.css';
import Counter from './components/Counter';
function App() {
const [thresholdReached, setThresholdReached] = useState(false);
const [count, setCount] = useState(0);
useEffect(() => {
if (count === 10) {
setThresholdReached(true);
}
if (count < 10) {
document.title = `Current Count: ${count}`;
}
}, [count]);
const increment = () => {
setCount(prevState => prevState + 1);
};
return (
<div className="App">
{thresholdReached ? (
<p>You've reached the count threshold! ;)</p>
) : (
<Counter
count={count}
increment={increment}
/>
)
}
</div>
)
}
export default App;
As you might expect, we have the state initialization for the count which is used to supply the Counter element we are conditionally rendering based on the computed thresholdReached state value, as well as an useEffect hook that’s used to track any changes to the count state variable.
As long as the count value is lesser than 10 we’ll update the Browser Tab title to follow the “Current count: X” template; otherwise, we’ll unmount the component using the thresholdReached state variable and update the Browser Tab title to be simply “Counter”.
Demo
Summary
In this article, we’ve been able to go over the main concepts of lifecycle methods, understand what the useEffect hook is used for within React’s ecosystem, the ways we can make use of the 3 available lifecycle methods it provides us with, and also go over a small project in order to have a better understanding of how it would be used in a more real-life scenario.
Hopefully, you’ve got a better understanding of this concept and it help you in your future React projects.
In case I’ve omitted any important aspect, or you think I could’ve gone more over a specific part of the hook or any concept related to the hook, let me know by leaving a comment below.
See you at the next one. Cheers!
💬 Leave a comment