When we talk about persisting data of our application, most developers confuse their choice between cookies and local storage. Well, both of them serve different purposes and are different in their way. We are going to talk about both of them but keep our focus and implement cookies in this one.
What Are Cookies?
Well, I personally love the chocolate chip ones. 🤓 Anyhow, cookies are essential to websites in this new age, they make your personal experience better. It could be by
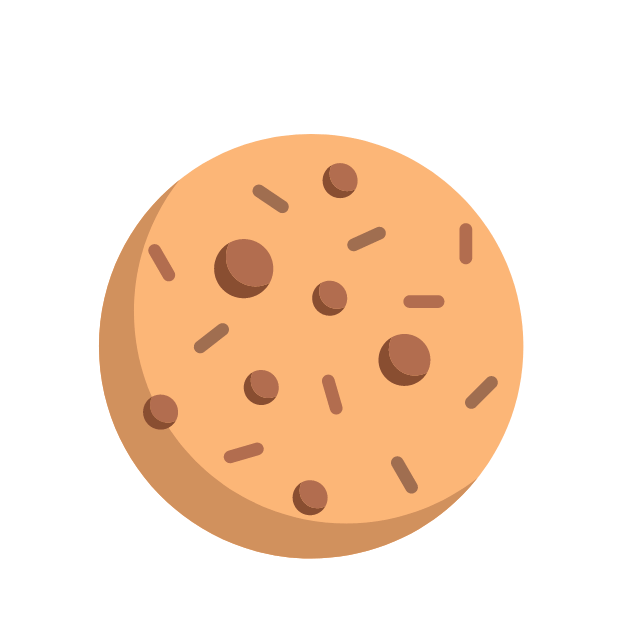
- Remember you as a user so that you don’t have to introduce yourself every time.
- Show what you want to see as a user
- Track your preference and much more
But they could also give piggyback rides to vulnerabilities or expose your data as well. There can be two types of cookies when you visit a website, they can be first party or third party. First-party cookies are the ones from the website you are using whereas third-party cookies are not from the current web page you are surfing, they could be from ads or some other parties trying to get some of your data.
Cookies in ReactJS?
You can have first-party cookies in your reactJS web application and let us see them in action.
npm i react-cookie
Once you have installed the package, we will start with wrapping our entire application with the cookie provider assisted by react-cookie
import React from "react";
import { CookiesProvider } from "react-cookie";
import ReactDOM from "react-dom/client";
import App from "./App";
import reportWebVitals from "./reportWebVitals";
const root = ReactDOM.createRoot(document.getElementById("root"));
root.render(
<React.StrictMode>
<CookiesProvider>
<AnyOtherProvider>
<App />
</AnyOtherProvider>
</CookiesProvider>
</React.StrictMode>
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
Once you have your provider configured, it is now time to see the real action
Inside you component
import { useCookies } from "react-cookie";
function CustomComponent() {
const [cookies, setCookie, removeCookie] = useCookies(["user"]);
const onSubmit = (data) => {
setCookie("user", data, { path: "/" });
};
return (
... rest of your component
<button
onClick={() => {
alert(`User cookie is ${JSON.stringify(cookies["user"])}`);
}}
>
Show user cookie
</button>
<button
onClick={() => {
removeCookie("user");
}}
>
Delete user cookie
</button>
)
}
Here we are using useCookies hook by react-cookie, which helps us clean set and get our cookies, so I have basically integrated this functionality into one of our existing forms tutorials with the addition of two buttons that alert the current cookie and the other which removes the cookies.
Moment Of Truth 🤩
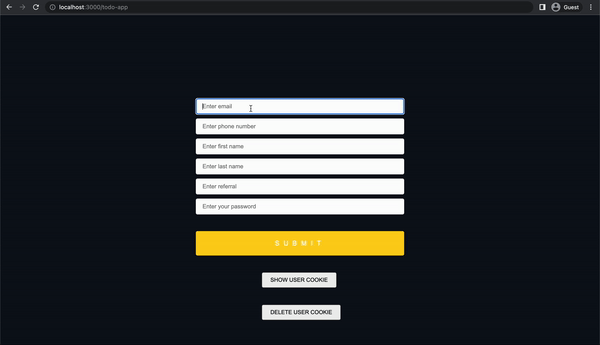
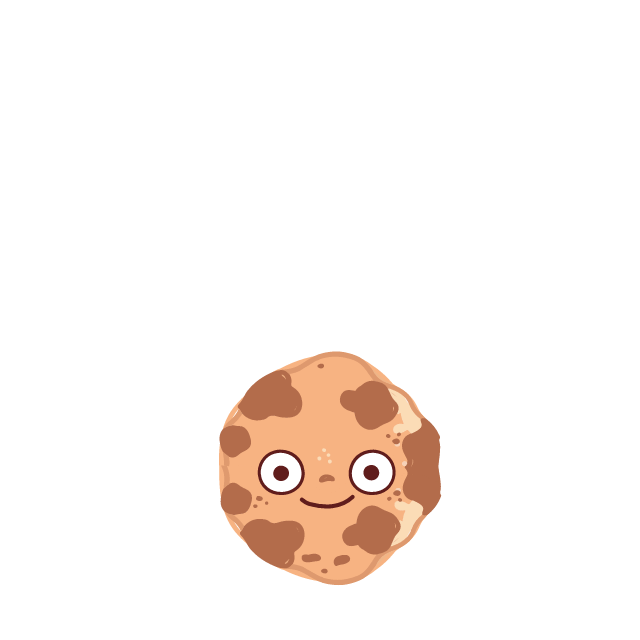
Wrap Up
We have talked about the different ways you can utilize cookies in your react application. Whether it is with Session Management, Personalisation, Tracking, or Authentication. And we learned the easiest way to get started with cookies in your reactjs application. That is it for this one, see you at the next one. Take care.
💬 Leave a comment