Comments are really useful when it comes to documenting code. As a rule of thumb, a well-written piece of software shouldn’t be using comments as the variable and function names should be self-explanatory.
However, it rarely happens in practice. Comments are quite useful when it comes to explaining why are we doing things the way we do. They can be helpful not only to other engineers but also to yourself.
Test App
I have written a simple application that we’ll use to demo commenting.
const App = () => {
console.log("My name is Tommy")
return (
<div style={{ margin: '50px' }}>
<h1>My names is:</h1>
<h2>Tommy</h2>
</div>
)
}
export default App;
This app renders a simple string to the page and logs it to the console.

JSX
As you probably know, JSX is not HTML. For that reason, we cannot use the HTML comments inside JSX. We’ll need to use the JS comments.
You’ll need to use the /* … */ syntax enclosed in the curly braces { }. It should look like this
{/* Comment */}
Let’s take a look at the code example.
const App = () => {
console.log("My name is Tommy")
return (
<div style={{ margin: '50px' }}>
<h1>My names is:</h1>
{/* <h2>Tommy</h2> */}
</div>
)
}
export default App;
As expected, the h2 element disappears from the page.
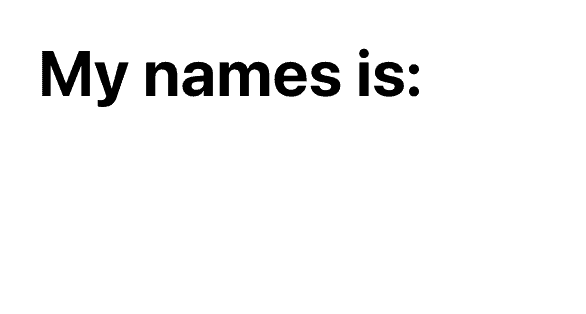
Component body
To comment in the component body you should be using the // syntax.
// Comment
Let’s take a look at the app example.
const App = () => {
// console.log("My name is Tommy")
return (
<div style={{ margin: '50px' }}>
<h1>My names is:</h1>
<h2>Tommy</h2>
</div>
)
}
export default App;
Now go ahead and check the console if the console.log statement runs.
Shortcuts
Undoubtedly the easiest way to comment your code in React project is to use the editor shortcut. If you’re using the VS Code like me then all you have to do is to select the piece of code that you wish to comment out and then use the shortcut. Those are:
- Command + / on MAC
- CTRL + / on Windows
💬 Leave a comment