It’s actually quite simple to use images in React applications. Let’s take a quick look at how it works.
Passing the URL
The simplest way would be to do it just like in a regular web application by passing the relevant URL string to the src property of the HTML image tag. Let’s use the below URL as a guide.
https://reactjs.org/logo-og.png
function App() {
return (
<div style={{ margin: '100px' }}>
<img src="https://reactjs.org/logo-og.png" alt="react logo" style={{ width: '400px', }}/>
</div>
);
}
export default App;
This should render the following to the user.
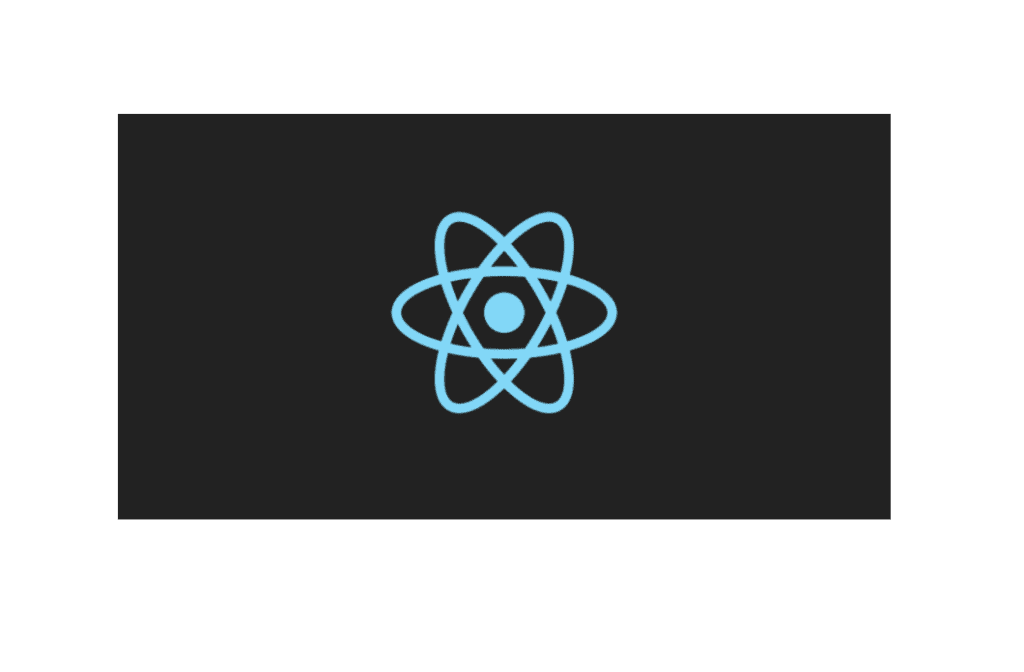
Modules
You can import an image via modules just like you would be importing regular components. Importing an image this way generates a string value, which can later be used in your JSX. You can now use this value and pass it to the src property of the image HTML tag.
In this case, the image must be located somewhere in the src directory of your React project. It is a good practice to group all such files in a subdirectory called assets.
Go ahead and create such a directory and place there an image that you’d like to use. In my case, it’s going to be a picture of Harry Potter called harry-potter.jpg
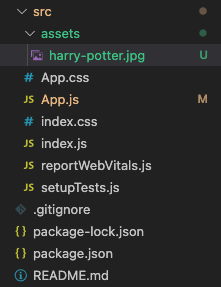
We can then import it into our application and use it as follows:
import HarryPotterImage from './assets/harry-potter.jpg'
function App() {
return (
<div style={{ margin: '100px' }}>
<img src={HarryPotterImage} alt="harry potter" style={{ width: '400px', }}/>
</div>
);
}
export default App;
Which should then render the below image.
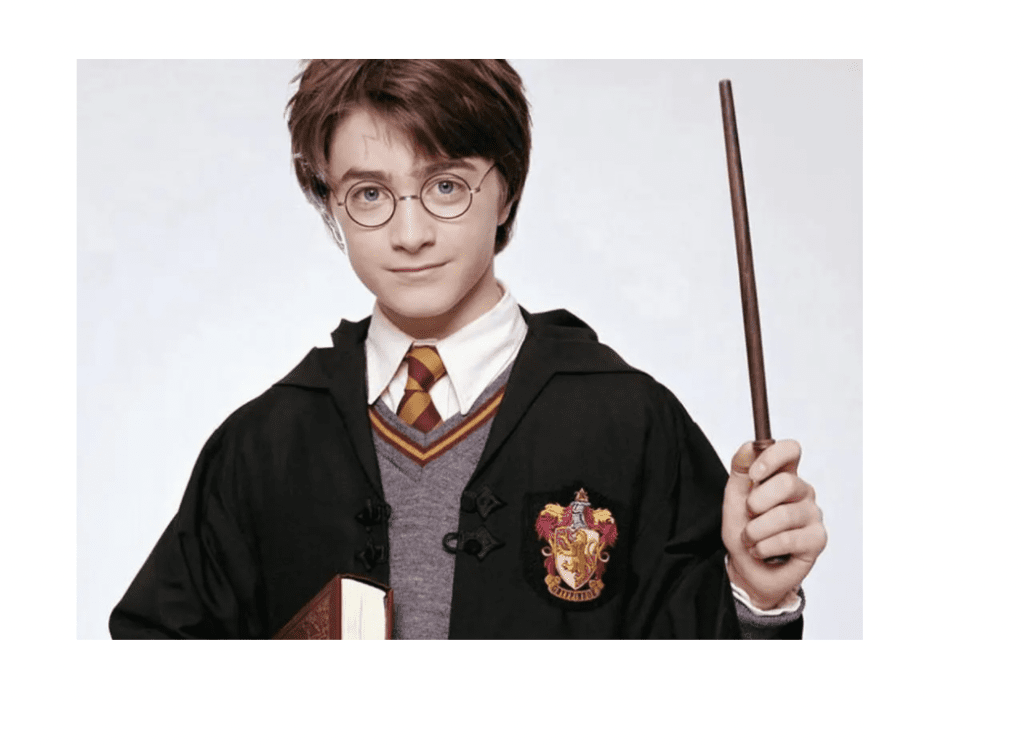
It’s worth mentioning that for accessibility reasons you should never skip adding the alt property to the HTML image tag. The text provided will be used in case the image fails to load. Some web users are relying on screen readers to read the text from the alt property.
Alternatively, you may be interested in using imaged with the CSS property background-image. This topic has been covered by Jesse in his article on how to use background images with React.
💬 Leave a comment