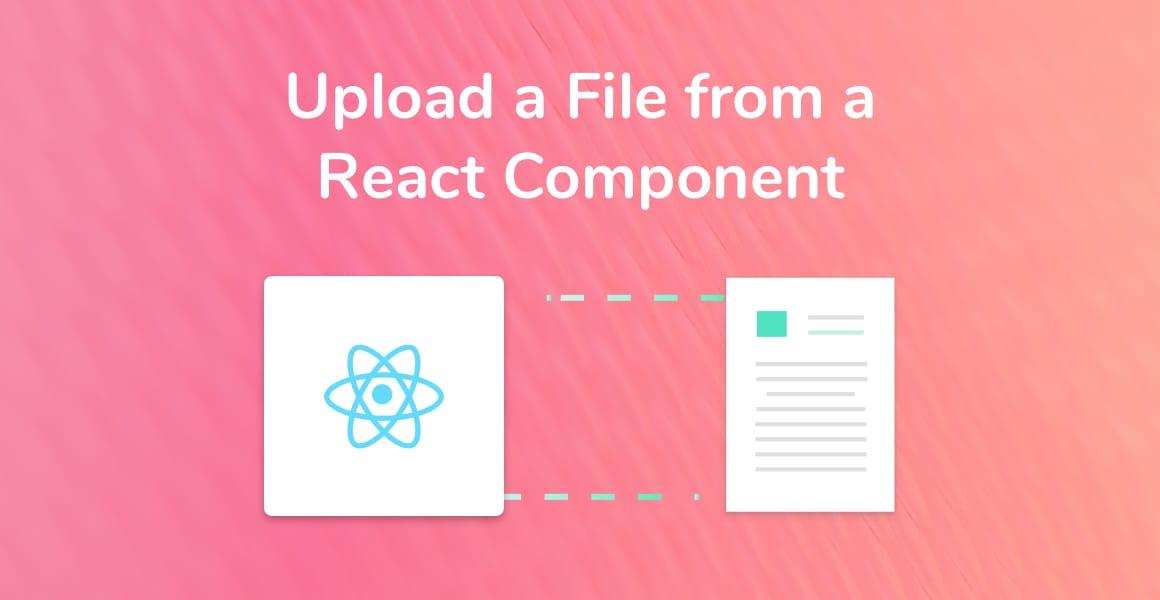
There are multiple ways to upload a file using React. I’m going to explain the steps to upload a single file, multiple files, and files and data in React.
We’re going to cover every scenario you might come across to upload a file from inside of a React component. These are:
How to Upload a File in React
We’ll start with the easiest and most common scenario, which is how to upload a single file to a server from a React component.
If you want to learn how to create a drag-and-drop file upload component in React, check out React Dropzone and File Upload in React.
Any type of file upload in React, or any front-end JavaScript library for that matter, requires an HTTP library to send the file data to a server.
I’m using the Fetch HTTP library in the following examples, but you can easily adapt them to work with other HTTP libraries like Axios or SuperAgent.
function uploadFile(file) {
fetch('https://path/to/api', {
// content-type header should not be specified!
method: 'POST',
body: file,
})
.then(response => response.json())
.then(success => {
// Do something with the successful response
})
.catch(error => console.log(error)
);
}
The example above uses a function, uploadFile, which takes a file object and passes that object to a POST request.
There are two things you need to remember when POSTing a file object:
- Never set the Context-Type Header.
- Pass the whole file object (Blob) to the body of the request.
Content-Type and Uploading a File
If you’ve made API requests in the past, then you probably used “application/
You do not need to set the content-type header when sending a file in a POST request.
File objects (Blobs) are not JSON, and therefore using an incorrect content-type will cause the request to fail. Web browsers automatically set the content-type header when sending a file in a POST request.
How to Upload Multiple Files in React using FormData
When we need to upload multiple files using Fetch, we have to use a new type of object called FormData.
FormData allows us to append multiple key/value pairs onto the object. After we’re done appending, we then pass it to the POST request’s body.
Let’s see an example of this below:
function uploadFile(files) {
var formData = new FormData();
files.map((file, index) => {
formData.append(`file${index}`, file);
});
fetch('https://path/to/api', {
// content-type header should not be specified!
method: 'POST',
body: formData,
})
.then(response => response.json())
.then(success => {
// Do something with the successful response
})
.catch(error => console.log(error)
);
}
How to Upload Multiple Files and Other Data in React
There may be times when you need to send files and other data in a POST request. This can sometimes be referred to as multipart data.
For example, you may have a form which asks for a user’s first and last name, as well as a profile picture for their account avatar.
In this case, you’d want to use FormData again to send the file as well as the first name and last name. Let’s see how we’d use FormData to help us send all of this data together:
function uploadFile(files, user) {
var formData = new FormData();
files.map((file, index) => {
formData.append(`file${index}`, file);
});
formData.append('user', user);
fetch('https://path/to/api', {
// content-type header should not be specified!
method: 'POST',
body: formData,
})
.then(response => response.json())
.then(success => {
// Do something with the successful response
})
.catch(error => console.log(error)
);
}
We’re simply using the formData.append method to create another key named user, and then passing the user object into the value of that key.
Thank you for following along and if you are new to React, don’t forget to check out this article to learn how to create your first React app.
💬 Leave a comment