Chakra UI is a popular library for building user interfaces with a focus on accessibility and developer experience. It provides a set of reusable components that can be easily integrated into any project. In this article, we will go over the process of setting up Chakra UI with Next.js, a popular framework for building server-rendered React applications.
Creating your Next.js app
If you’re adding Chakra UI to an existing app, you can feel free to skip this step.
First, we will need to create a new Next.js project. We can do this by running the following command in our terminal:
npx create-next-app my-app
# or with TypeScript
npx create-next-app@latest --ts
This will create a new directory called “my-app” with the basic structure of a Next.js project. Feel free to swap out “my-app” for whatever your app should be called. I also tend to use TypeScript with my Next.js apps, but you can choose either TS or JS.
Setting Up Chakra UI
Next, we will need to install Chakra UI and its dependencies. We can do this by running the following command in our terminal:
npm install @chakra-ui/react @emotion/react @emotion/styled framer-motion
Example
Let’s showcase Chakra UI by building a simple social card. Here’s what the end result will look like:
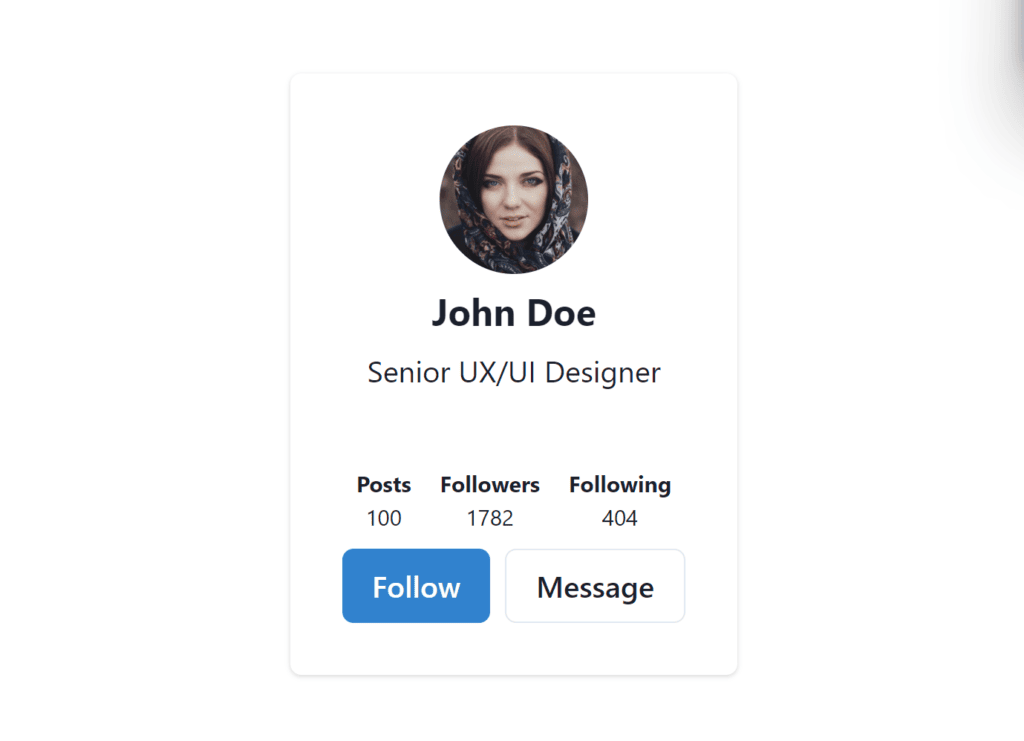
Firstly, to make sure that the Chakra UI styles are applied globally, we need to wrap our application in the ChakraProvider component.
import { ChakraProvider } from '@chakra-ui/react';
export default function MyApp({ Component, pageProps }: AppProps) {
return (
<ChakraProvider>
<Component {...pageProps} />
</ChakraProvider>
);
}
Here’s the code for our page component:
export default function Home() {
return (
<Flex w="100vw" h="100%" align="center" justify="center">
<Head>
<title>Hello World!</title>
<link rel="icon" href="/favicon.ico" />
</Head>
<main>
<Flex w="100%" h="100vh" align="center">
<ProfileCard />
</Flex>
</main>
</Flex>
);
}
We’re starting off with our main page component. Chakra UI provides a generic Flex component, which gives us a generic container with “display: flex” applied. Chakra UI works around style props, which let you provide values through props. There are a lot of shorthand versions of these props, such as “w” instead of “width”, “align” instead of “alignItems”.
Let’s drill into the ProfileCard component:
function ProfileCard() {
return (
<Card
w="auto"
display="flex"
direction="column"
align="center"
justify="center"
padding="2">
<ProfileHeader />
<ProfileBody />
</Card>
);
}
I’m using ChakraUI’s generic card component. This is a generic card component with a rounded edge and shadow. One helpful part is that ChakraUI allows you to split your card up into parts, e.g. CardHeader, CardBody, and CardFooter.
Then inside our header:
function ProfileHeader() {
return (
<CardHeader
display="flex"
flexDirection="column"
gap="2"
alignItems="center">
<Avatar
height="80px"
width="80px"
src="https://i.pravatar.cc/300"
/>
<Heading size="md">John Doe</Heading>
<Text>Senior UX/UI Designer</Text>
</CardHeader>
);
}
Again I’m using style props to pass through styles. I’m also using the ChakraUI Avatar component, used to represent users/profile pictures.
Inside the body:
function ProfileBody() {
return (
<CardBody display="flex" flexDirection="column" gap="2">
<ProfileStats />
<ProfileButtons />
</CardBody>
);
}
We have a stats and buttons component, so let’s break these down.
In the stats:
function ProfileStats() {
const stats = {
posts: 100,
followers: 1782,
following: 404,
};
return (
<HStack w="100%" justify="space-evenly">
{Object.entries(stats).map(([title, value]) => (
<Flex
fontSize="xs"
key={title}
justify="center"
align="center"
direction="column">
<Text casing="capitalize" fontWeight="bold">
{title}
</Text>
<Text>{value}</Text>
</Flex>
))}
</HStack>
);
}
We have an HStack component, used for horizontally stacking items. ChakraUI also provides VStack for vertical stacks, as well as Stack, which can take a direction as a prop.
Then in the ProfileButtons:
function ProfileButtons() {
return (
<ButtonGroup colorScheme="blue">
<Button>Follow</Button>
<Button variant="outline">Message</Button>
</ButtonGroup>
);
}
ChakraUI provides some pre-built button styles which are very helpful, with a variety of colours and variations. It also provides a built-in ButtonGroup component, which has the added benefit of sharing styles between buttons.
And that’s all of our components from start to finish!
Conclusion
And that’s it! We have successfully set up Chakra UI with Next.js. With this setup, you can start using Chakra UI’s pre-built components, and styles to quickly build accessible, and visually pleasing interfaces.
Remember to also check the documentation for the library and the framework, to see all the features and possibilities. We also have articles covering alternative styling solutions, such as TailwindCSS.
If you liked this article, feel free to leave a comment below!
Happy coding!
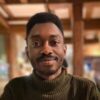
💬 Leave a comment