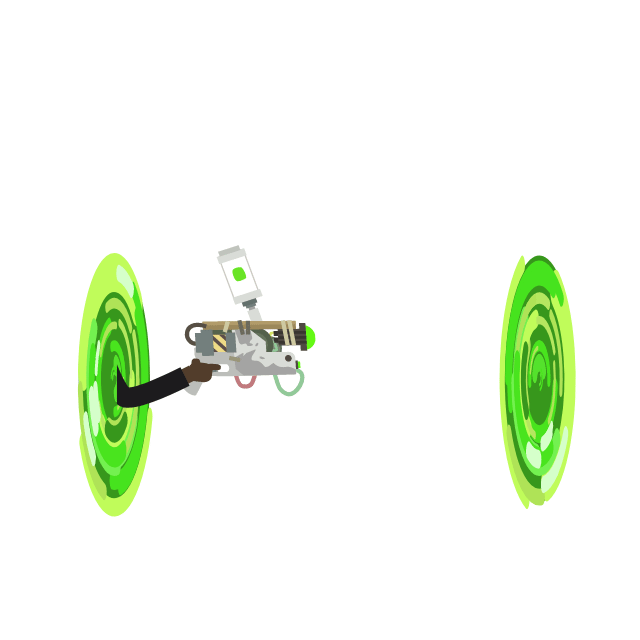
Introduction
In this article, we are going to render child components, which are outside the Document Object Model(DOM) to dwell within the DOM 🤯. In simpler terms, to make use of a component that exists outside the DOM hierarchy of the parent component.
Usage
Portals can be used as
- Dialog Boxes
- Modals
- Tooltip
- Loaders
Why Use Portals?
A react portal acts as a normal react component wherever you plug it in and has all the abilities and power as any other react component. Here are some advantages of react portals
- Event Bubbling: With a portal that is situated outside the DOM tree, it can still communicate with the parent component as a normal child component do.
- With Context: Portals can also use context to get/set data.
Portal In Action
import React from "react";
import ReactDOM from "react-dom";
import styles from "./Modal.module.css";
const PortalComponent = ({ setIsOpen }) => {
return ReactDOM.createPortal(
<>
<div className={styles.darkBG} onClick={() => setIsOpen(false)} />
<div className={styles.centered}>
<div className={styles.modal}>
<div className={styles.modalHeader}>
<h5 className={styles.heading}>Portal</h5>
</div>
<div className={styles.modalContent}>
This is a portal, not in the scope of the parent component but still
in the DOM tree 🤩🤯
</div>
<div className={styles.modalActions}>
<div className={styles.actionsContainer}>
<button
className={styles.deleteBtn}
onClick={() => setIsOpen(false)}
>
Yay! 🎉🥳
</button>{" "}
</div>
</div>
</div>
</div>
</>,
document.body
);
};
export default PortalComponent;
We have created a portal with ReactDOM.createPortal() which returns our portal component. We have compiled this component exactly how a normal functional component would return a component. And now we can plug it in anywhere in our code and see the magic happen.
const CustomComponent = () => {
const [openPortal, setOpenPortal] = useState(false);
return (
<div>
<button
className="button"
onClick={() => setOpenPortal(!openPortal)}
>
Open Portal Modal
</button>
</div>
)
}
export default CustomComponent
We have plugged in the portal with our custom component and managed its state from the component, similar to parent/child communication.
Moment Of Truth
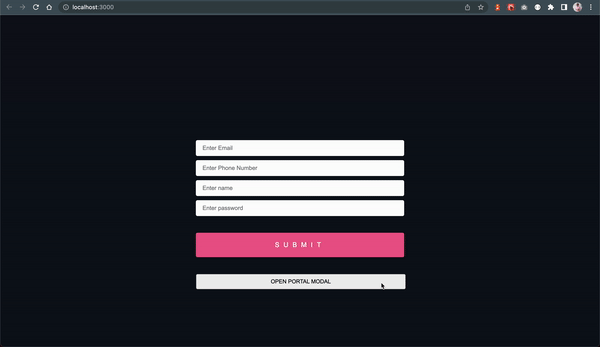
Wrap Up
Wow! That is MAGIC! 🪄 I hope you learned a lot from this article, trying to explain this little but valuable concept in reactJS. You can also inspect and see for yourself that the modal is indeed outside of the root node of the application. I’ll see you at the next one. Take care.
💬 Leave a comment